字符串类算法笔记
1.KMP算法笔记
以下为2篇kmp详解链接
https://leetcode-cn.com/leetbook/read/array-and-string/cpoo6/
https://blog.csdn.net/qq_45806146/article/details/104911112?spm=1001.2101.3001.6650.1&utm_medium=distribute.pc_relevant.none-task-blog-2%7Edefault%7ECTRLIST%7ERate-1.pc_relevant_default&depth_1-utm_source=distribute.pc_relevant.none-task-blog-2%7Edefault%7ECTRLIST%7ERate-1.pc_relevant_default&utm_relevant_index=2
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| #include <iostream> #include "string" #include "vector" using namespace std; class Solution { public: vector<int> next; void buildNext(string s) { int m = s.size(), j = 0; int t = -1; next.resize(m); next[0] = t; while (j < m - 1) { if (t < 0 || s[j] == s[t]) { j++; t++; next[j] = t; } else { t = next[t]; } }
} int strStr(string haystack, string needle) { if (needle.size() == 0) return 0; buildNext(needle); int n = haystack.size(), i = 0; int m = needle.size(), j = 0; while (i < n && j < m) { if (j < 0 || haystack[i] == needle[j]) { i++; j++; } else { j = next[j]; } } if (j == m) { return i - j; } return -1; } };
|
2.寻找旋转排序数组中的最小值
二分查找
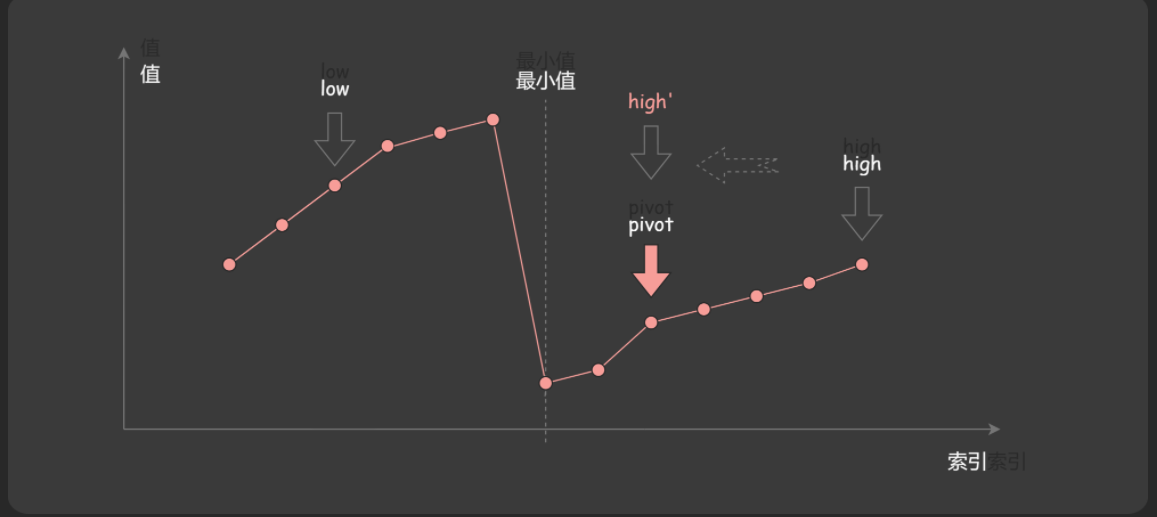
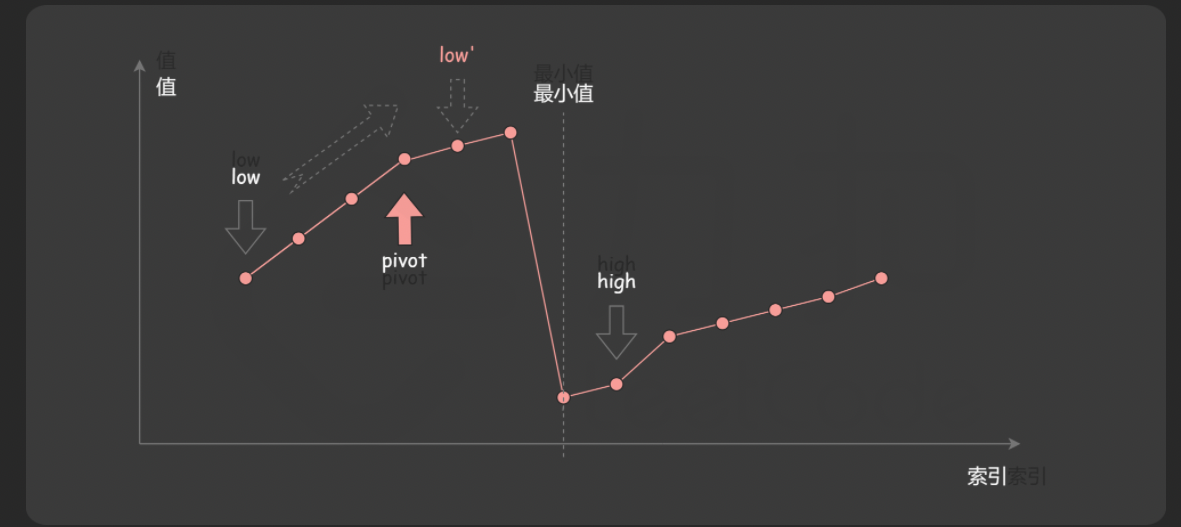
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| int findMin(vector<int>& nums) { int high = nums.size()-1; int low = 0; int mid; while (low<high) { mid = (low+high)/2; if(nums[mid]<nums[high]){ high = mid; }else{ low = mid + 1; } } return nums[low]; }
|